Drawing a 2D house with JavaScript
I share how to create a Javascript Canvas 2D house without using external libraries and what I learned by following the CanvasRenderingContext2D docs.
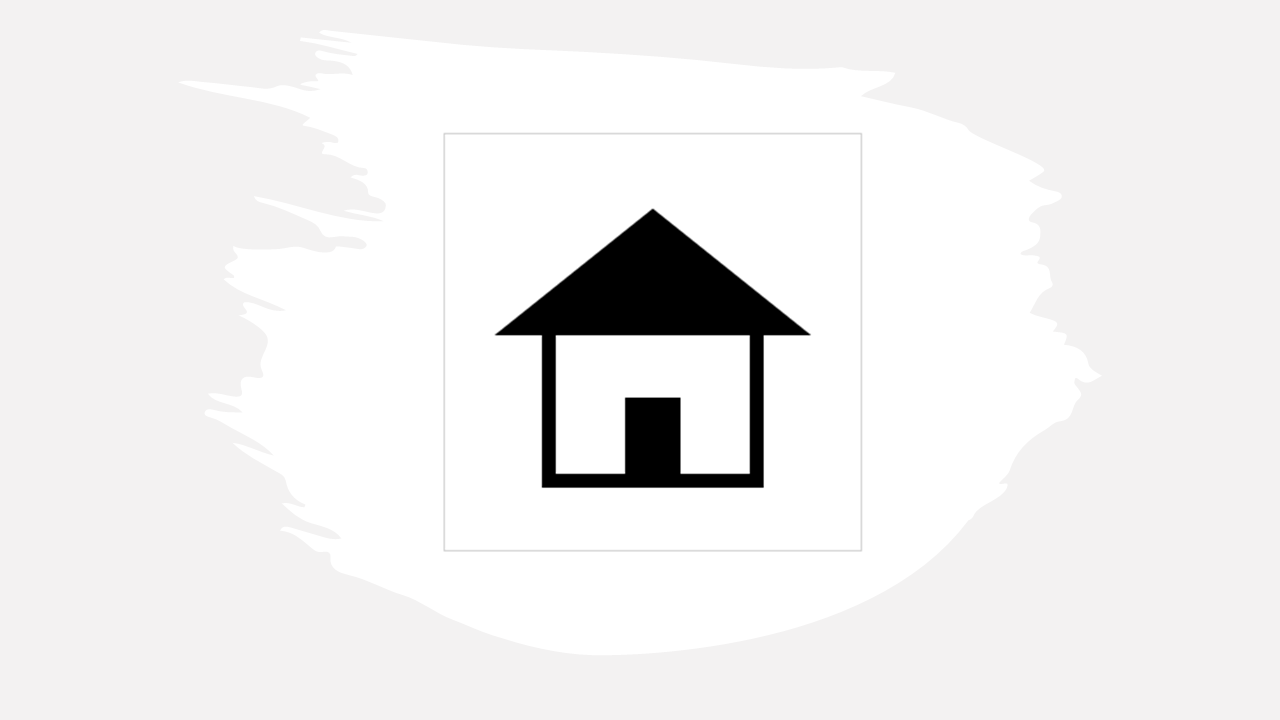
The end result
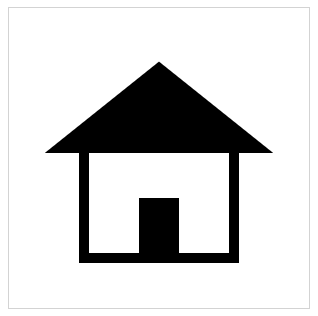
Code
<!DOCTYPE html>
<html>
<head>
</head>
<body>
<canvas id="my-house" width="300" height="300" style="border:1px solid #d3d3d3;"></canvas>
<script>
var canvas = document.getElementById("my-house");
const ctx = canvas.getContext('2d');
ctx.lineWidth = 10;
//Wall
ctx.strokeRect(75, 140, 150, 110); //x, y, width, height
//Door
ctx.fillRect(130, 190, 40, 60);//x, y, width, height
//Roof
ctx.beginPath();
ctx.moveTo(50, 140);// left most point
ctx.lineTo(150,60); // line to top point
ctx.lineTo(250,140); //line to right most point
ctx.closePath(); // links starting point and end points
ctx.stroke(); // adds a stroke to the invisible path
ctx.fill(); // adds a fill to the path
</script>
</body>
</html>
Note that I merely modified the example from the Official docs and added some notes.
What I learned
Path
When using paths to draw triangles, if one already has 2 strokes, manually adding the 3rd stroke is not needed. Closing the path automatically adds the last stroke by connecting the path's starting and end points with a straight line.
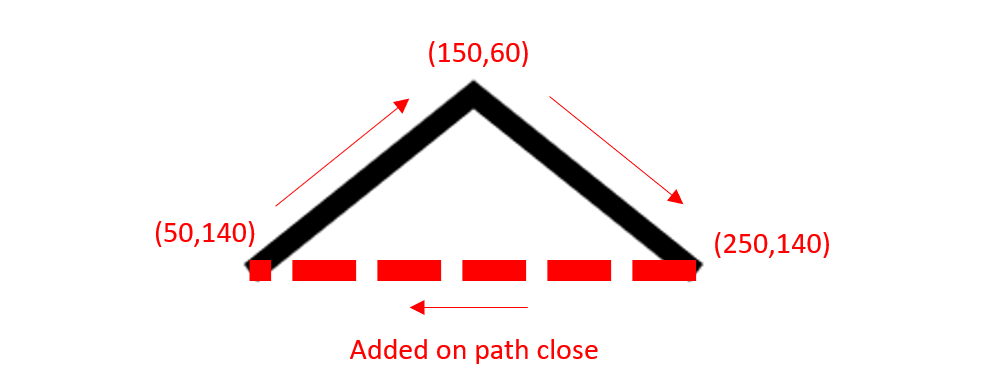
Also, if one does not manually add a stroke/fill to a path nothing will be displayed.
Fill
Adding only a fill (no stroke) will result in a smaller shape than expected.
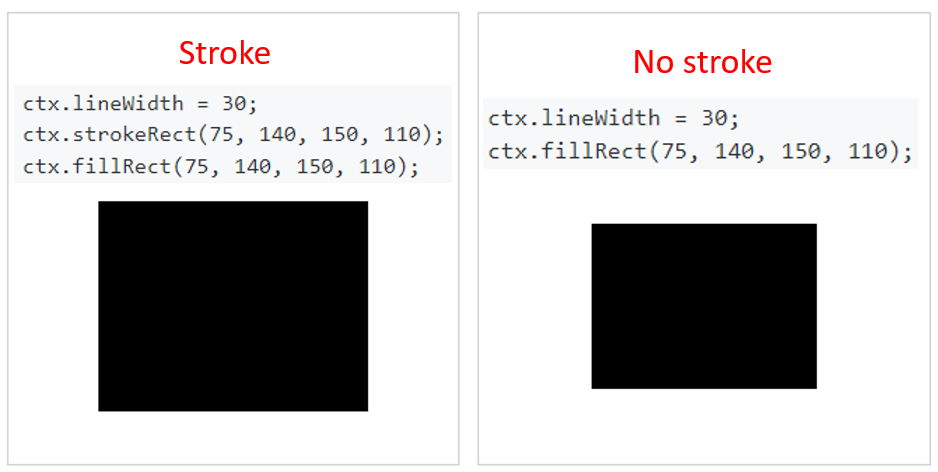