JavaScript basics
Examples of different JavaScript syntax examples based on ES6+.
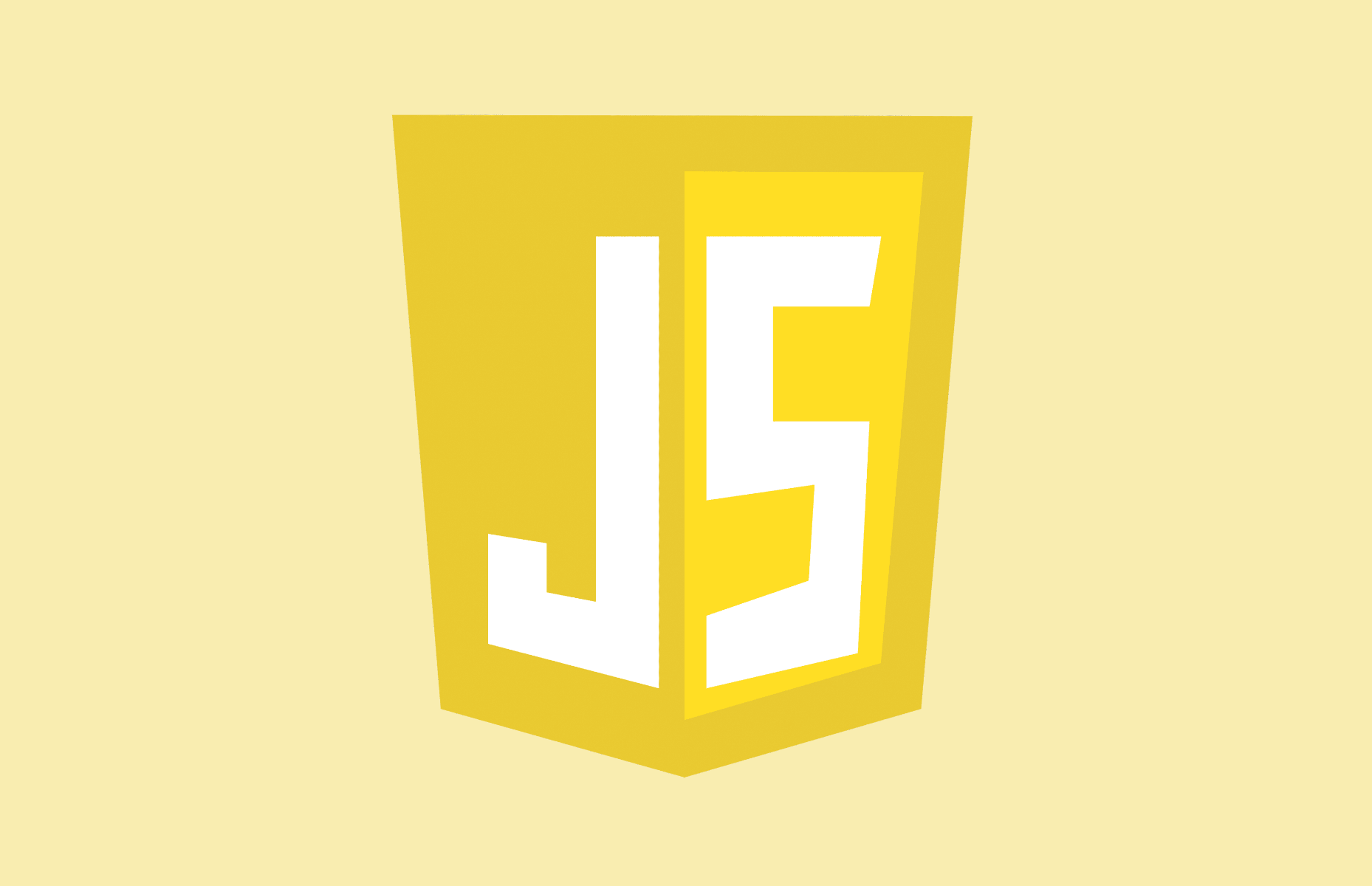
Variable
Declaration | Scope | Mutable assignment |
---|---|---|
var | function | true |
let | block | true |
const | block | false |
Function
//Option 1
let product = function(i, j) {
return i * j;
}
//Option 2
let product = (i, j) => i * j;
//Option 3
let product = (i, j) => {
return i * j;
}
Class
Option 1:
//Declaring a class:
function SomeClass(someVar) {
this.someVar = someVar;
}
//Adding a function to a class:
SomeClass.prototype.someFunction = function(){
//Some action
}
//Instantiating an object
const someItem = new SomeClass(someVar);
someItem.someVar;
someItem.someFunction();
Option 2:
class SomeClass {
constructor(someVar){
this.someVar = someVar;
}
someFunction(){}
}
Inheritance:
class Child extends Parent {
constructor(someVar){
super(someVar);
}
someOtherFunction(){}
}
No concept of interfaces.
Module
In module.js:
export function someFunction(){}
let someVar = "";
let someSecondVar = "";
export {someVar, someSecondVar};
Import in some other file:
import {someFunction, someVar, someSecondVar as someOtherVar};
//variables will be readonly
Array, Set, Hashmap
Array:
const a = [];
const b = new Array();
Set:
const a = new Set();
Hashmap
const a = new Map();
Promise
//Creating a promise
function someFunction(message) {
return new Promise((resolve, reject) => {
setTimout(()=> {
try {
console.log(message);
resolve();
}
catch(e) {
reject(e);
}
}, 1000);
});
}
//Calling it after one another
someFunction("1st call")
.then("2nd call")
.then("3rd call")
.catch(function(e){
console.log(e);
});
Async and await
The async function allows the await keyword in its body, avoiding chained promise calls:
async function someFunctionManyTimes() {
try {
await someFunction("1st Call");
await someFunction("2nd Call");
await someFunction("3rd Call");
} catch (e) {
console.error(e.message);
}
}
someFunctionManyTimes();
The following are similar:
async function someFunction() {
return "a";
}
function someFunction() {
return Promise.resolve("a");
}