TypeScript basics
Some TypeScript code examples for quick reference.
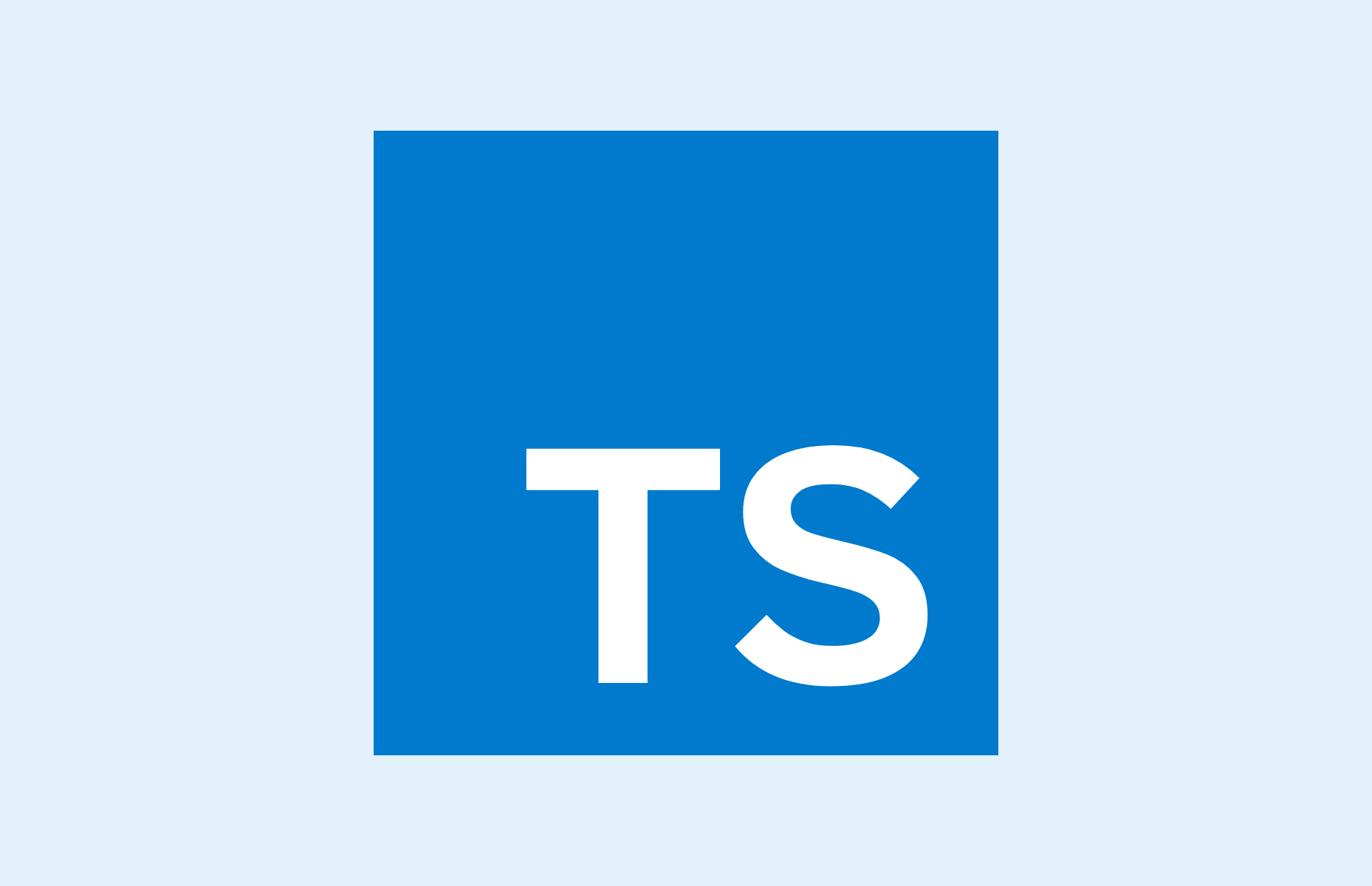
What is it?
Developed by Microsoft, it adds static typing to JavaScript.
Pros
- IDE code completion (e.g. IntelliSense)
- IDE error indicators on more cases.
- Adds interfaces and enums
Cons
- Level of entrance: Learn more on top of JavaScript, although it helps that most of the JavaScript syntax still works as is.
- Can add extra complexity
- Reduced build performance to compile TS to JS
tsconfig.json
TypeScript settings. Especially compilation settings e.g. which ES version to compile to.
Basic types
- Boolean
- Number
- String
- Array
- Tuple
- Enum
- Unkown
- Any
- Void
- Null and undefined
- Never
- Object
Tuple
An array with a fixed number of elements whose types are known:
let someTuple: [number, string] = [1, "someString"];
Enum
enum SomeEnum {
ONE = '1',
TWO = '2',
}
Interface
Example:
interface SomeInterface {
someProperty1: string;
readonly someProperty2: string;
someFunction1: () => string;
someFunction2?: () => string;
}
Note that the '?' causes a property/method to be optional.
Implementation:
//Option 1:
let someObject: SomeInterface = {
someProperty1: "property1",
someProperty2: "property2", //won't be able to change this afterwards
someFunction: () => "someString";
}
//Option 2:
class SomeClass implements SomeInterface {
someProperty1: string;
readonly someProperty2: string;
constructor(someProperty1: string, someProperty2: string){
this.someProperty1 = someProperty1;
this.someProperty2 = someProperty2;
}
someFunction1() {
return "someString";
}
}
//Option 3 (Readonly):
let someUnreadableObject: Readonly<SomeInterface> {
someProperty1: "property1",
someProperty2: "property2",
someFunction: () => "someString";
}
Interfaces can also extend other interfaces.
Array
let a: string[] = ["a", "b", "c"];//prefered method
let a<string> = ["a", "b", "c"];
//multiple types - not the cleanest practice, but possible
let a: (string|number)[] = ["a", 1, "b"];
Unkown
Introduced in TypeScript 3. Recommended to always use it instead of unknown.
let someAny: any = 1;
let someUnknown: unknown = 1;
let someString: string = someAny; //works
let someString2: string = someUnknown; //error
let someString3: string = someUnknown as string; //works
Assertion
When you tell typescript to treat your variable as a different type. Example:
let someValue: unknown = "SomeText";
let length = (someValue as string).length;
Void
The type void means nothing. Example:
const someFunction = ():void => {
//does something
}
Thus above, nothing is returned.
Elvis operator, ?? and ||
return someObject?.someProperty ?? ''; //if someObject if defined it will return someProperty and if someProperty is null or undefined it will return ''
return count || ''; // the || operator checks if count is falsy (null, undefined, false, 0, NaN and empty strings)
Pick and omit
Let's say you have the following interface:
interface User {
name: string,
email: string,
password: string
}
How can we create a new based on User without the name property?
type OptionOne = Pick<User, 'email' | 'password'>;
type OptionTwo = Omit<User, 'name'>;
Generic types
Allows to use something reusable with different datatypes.
function printObj<T>(obj: T) {
console.log("obj: " + T);
}
printObj("one");
printObj(1);